⏲️ Estimated reading time: 3 min
Learn how to create a real-time JavaScript Digital Clock that includes hours, minutes, seconds, and milliseconds. Perfect for projects needing precise time updates.
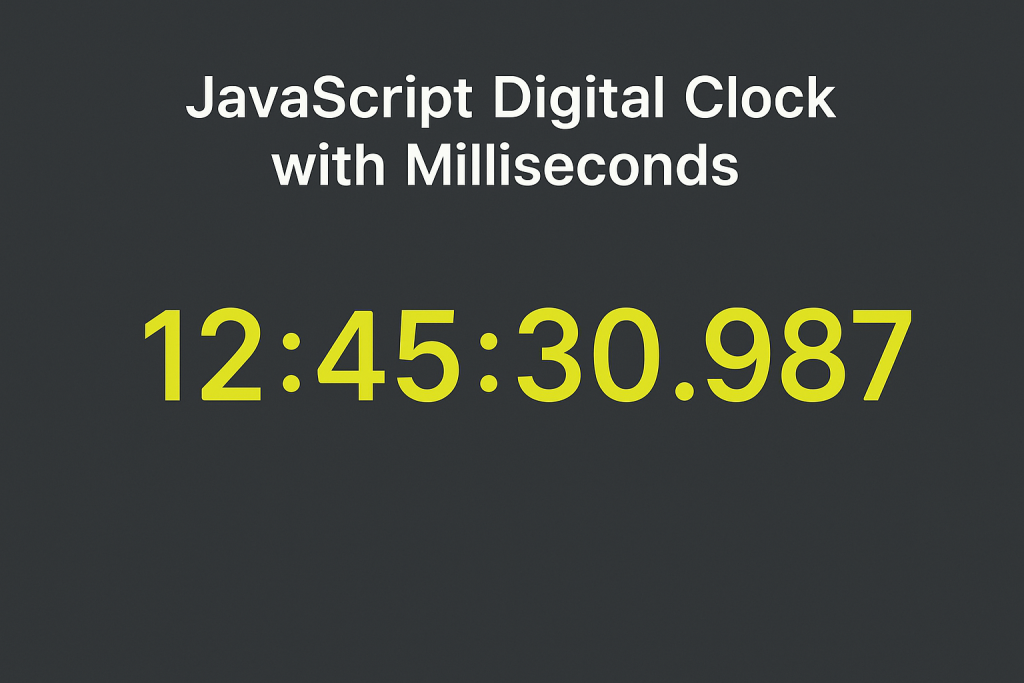
🕒 JavaScript Digital Clock with Milliseconds
Creating a digital clock that shows real-time updates including milliseconds is a fun and practical way to improve your frontend development skills. This JavaScript-based clock displays the current time with precision: hours, minutes, seconds, and even 3-digit milliseconds (000–999).
Whether you’re building a stopwatch, a countdown timer, a performance dashboard, or just want to learn more about handling dates and times in JavaScript, this small project is a perfect start. It updates the time every 50 milliseconds to ensure smooth and accurate display.
This digital clock shows real-time hours, minutes, seconds, and milliseconds, making it perfect for precision-focused web projects.
✅ How It Works
This clock uses JavaScript’s built-in Date
object to get the current time. It then formats the hours, minutes, and seconds to always appear with two digits, and the milliseconds are padded to three digits using padStart
.
Creating a real-time clock with milliseconds is a great way to visualize precise timing and improve UI interactivity. This tutorial shows you how to build one using plain JavaScript.
✅ HTML Setup
First, create a simple placeholder in your HTML:
<div id="clocktime"></div>
<body onload="startTime()">
✅ JavaScript Digital Clock Code
Use the following script to show current time including milliseconds, updated every 100ms:
<script>
function startTime() {
var today = new Date();
var h = today.getHours();
var m = today.getMinutes();
var s = today.getSeconds();
var ms = today.getMilliseconds();
h = checkTime(h);
m = checkTime(m);
s = checkTime(s);
ms = formatMilliseconds(ms);
document.getElementById("clocktime").innerHTML = "Today's current time is: " + h + ":" + m + ":" + s + ":" + ms;
setTimeout(startTime, 100); // Update every 100ms
}
function checkTime(i) {
return i < 10 ? "0" + i : i;
}
function formatMilliseconds(ms) {
ms = Math.floor(ms / 10); // Convert to 2-digit milliseconds (0-99)
return ms < 10 ? "0" + ms : ms;
}
</script>
✅ Why Use This Clock?
- Great for countdown features
- Ideal for live monitoring dashboards
- Enhances UI feedback in time-sensitive apps
- Stopwatch or timer apps
- Real-time performance metrics
- Training simulations
- Event countdowns
- Creative dashboards
This clock is lightweight, requires no libraries, and is fully customizable. You can tweak the update interval, change the time format, or even sync it with server time via API.
✅ Bonus Tip
If you want a smoother experience, you can style with CSS and even animate transitions for a sleek digital look.
🏷️ Tags: javascript, real-time updates, web development, coding tutorial, html, milliseconds, time display, frontend, beginner javascript
You want a downloadable zip, 👉 Click here to download js-digital-clock.zip
Only logged-in users can submit reports.
Discover more from HelpZone
Subscribe to get the latest posts sent to your email.